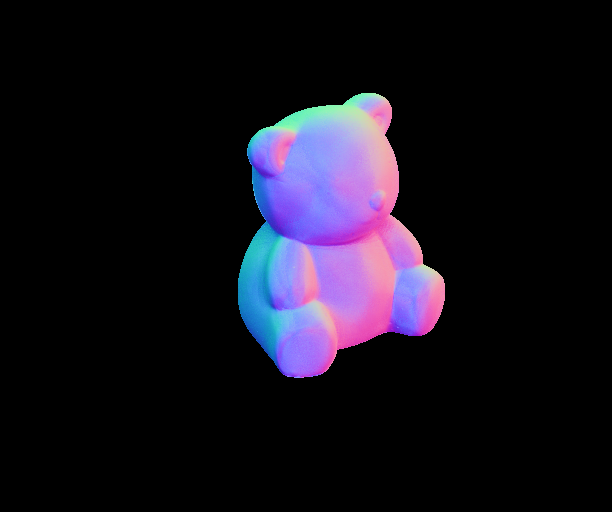
Photometric Stereo
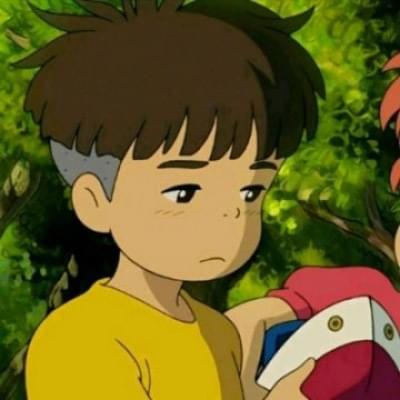
1. Experiment Purpose
The goal of this experiment is to implement the Photometric Stereo algorithm, which uses multiple images under different lighting directions to estimate the surface normal vectors and albedo, and then re-render the image under a specified lighting direction.
- Normal Vector Calculation: According to the Lambertian model formula
, where is the albedo, is the normal vector, and is the lighting direction. The albedo and lighting direction can be uniquely determined when at least three images with known lighting directions are provided. - Shadow and Highlight Processing: Shadows and highlights break the linear Lambertian model. A simple solution is to sort all the observations for each pixel and discard a certain percentage of the brightest and darkest pixels to remove shadows and highlights.
2. Experiment Principle
According to the Lambertian model (a purely diffuse reflection model), for each point on the surface, there is a fixed albedo
When we have images under different lighting directions, we can use the Lambertian model to analyze each pixel individually. With three lighting angles, we can express the Lambertian model equation in matrix form as:
With the albedo and normal vectors, re-rendering the image under a specified lighting direction is easy; we just apply the Lambertian model to calculate the light intensity at each pixel.
3. Experiment Content
In the provided code framework, the primary task is to implement the myPMS.m
file.
First, I modified the original function declarations because the final output requires three images: the normal map, albedo map, and re-rendered image, while the original framework only output the normal map. So, I added the albedo map and re-rendered image to the function's return. The input now also includes a new parameter shadow_removal_percentage
, which specifies the percentage of the brightest and darkest pixels to be discarded when handling shadows and highlights (e.g., if the value is 20, then the darkest 20% and the brightest 20% pixels are discarded).
In the data preprocessing phase, N
, albedo
, and re_rendered_img
represent the normal map, albedo map, and re-rendered image, respectively. They are all three-channel images. I
is used to temporarily store the light intensities for each channel.
Through simple loops, the original images were first processed with a mask
to extract the subject and avoid the influence of the background. Then, for each RGB channel, the given light_intensity
was used to divide the original image, retrieving the true light intensity I
for each channel.
Then, for each pixel, the light intensities of all the images were sorted, and the brightest and darkest pixels of the given percentage were discarded, storing the results in I_col_filtered
. Based on the formula s_filtered
and light intensities I_col_filtered
. The norm of the result gives the albedo value, and dividing the result by the albedo gives the unit normal vector.
With this information, re-rendering becomes straightforward. Simply traverse the pixels and apply the Lambertian model formula for each pixel. Since the assignment did not require the RGB components of the incident light, I assumed them to be 1 by default.
Thus, the main function for Photometric Stereo is complete. Below are some small modifications to the Baseline:
The function call now includes the modified inputs and outputs. The shadow and highlight removal percentage is set to 20, meaning the darkest 20% and the brightest 20% pixels are discarded. To make the final re-rendered image more visually appealing, it is also normalized.
Here is the full code for Photometric Stereo (expand to view):
(On my GitHub repository ComputerVision/Homework 1 you can also find the code)
Folding Click to view more
1 | function [N, albedo, re_rendered_img] = L2_PMS(data, m, shadow_removal_percentage) |
4. Experiment Results
Normal Map | Albedo Map | Re-rendered Picture | |
---|---|---|---|
bear | ![]() | ![]() | ![]() |
buddha | ![]() | ![]() | ![]() |
cat | ![]() | ![]() | ![]() |
pot | ![]() | ![]() | ![]() |
- 标题: Photometric Stereo
- 作者: Felix Christian
- 创建于 : 2024-12-09 14:18:05
- 更新于 : 2025-04-05 12:09:04
- 链接: https://felixchristian.top/2024/12/09/10-PhotometricStereo/
- 版权声明: 本文章采用 CC BY-NC-SA 4.0 进行许可。